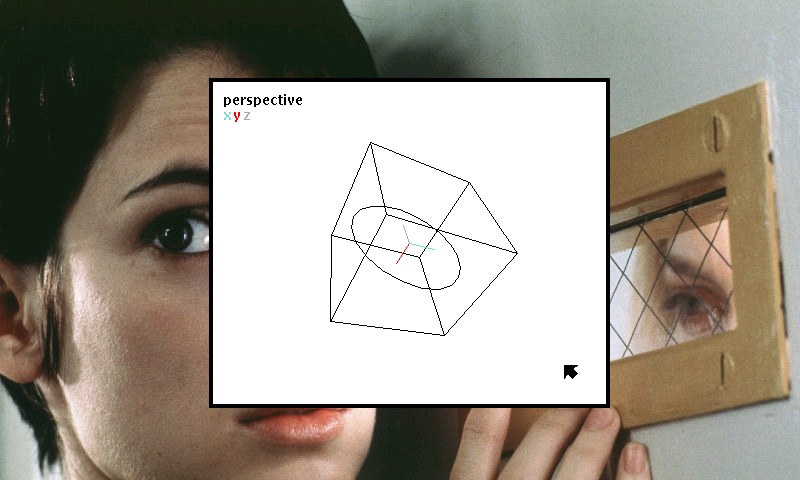
Moogle is a wireframe editor.
Moogle is a minimal 3D wireframe tool designed to be used alongside its companion tool Noodle, both can export to the icn format. It offers a handful of basic geometry drawing functions, it was written in ANSI C, and was inspired by Graf3DScene.
ANSI C
Creating wireframe scenes is done by modifying the program's source code.
cc -Os -DNDEBUG -g0 -s -Wall -L/usr/local/lib -lSDL2 -lm moogle.c -o moogle
Plan9 C
Moogle was first written on Plan9, the original Plan9 C implementation is available here:
5c moogle.c && 5l -o moogle moogle.5
Structures
Mesh * createdoorway(Scene *s, double width, double height, double depth, int color) { Mesh *doorway = addmesh(s); Point3d a = Pt3d(-width, 0, 0), b = Pt3d(-width, height, 0), c = Pt3d(0, height + width, 0), d = Pt3d(width, height, 0), e = Pt3d(width, 0, 0); addline(doorway, a, b, color); addline(doorway, b, c, color); addline(doorway, c, d, color); addline(doorway, d, e, color); addline(doorway, e, a, color); extrude(doorway, 0, 0, depth, color); return doorway; } Mesh * createstairs(Scene *s, double width, int steps, int color) { int i; Point3d a, b, c, d; Mesh *stairs = addmesh(s); for(i = 0; i < steps; ++i) { a = Pt3d(-width, i + 1, i), b = Pt3d(width, i + 1, i), c = Pt3d(-width, i + 1, 1 + i), d = Pt3d(width, i + 1, 1 + i); addline(stairs, a, b, color); addline(stairs, c, d, color); addline(stairs, a, c, color); addline(stairs, b, d, color); addline(stairs, a, Pt3d(a.x, a.y - 1, a.z), color); addline(stairs, b, Pt3d(b.x, b.y - 1, b.z), color); } addline(stairs, c, Pt3d(c.x, 0, c.z), color); addline(stairs, d, Pt3d(d.x, 0, d.z), color); addline(stairs, Pt3d(c.x, 0, c.z - steps), Pt3d(c.x, 0, c.z), color); addline(stairs, Pt3d(d.x, 0, d.z - steps), Pt3d(d.x, 0, d.z), color); return stairs; } Mesh * createring(Scene *s, double radius, double thickness, double depth, int segs, double angle, int color) { Mesh *ring = addmesh(s); /* front */ addarc(ring, radius, segs, angle, color); addarc(ring, radius + thickness, segs, angle, color); addedge(ring, ring->vertices, ring->vertices + segs + 1, color); addedge(ring, ring->vertices + segs, ring->vertices + segs * 2 + 1, color); /* back */ moveto(s, 0, 0, depth); addarc(ring, radius, segs, angle, color); addarc(ring, radius + thickness, segs, angle, color); addedge(ring, ring->vertices + segs * 3 + 2, ring->vertices + segs * 4 + 3, color); addedge(ring, ring->vertices + segs * 2 + 2, ring->vertices + segs * 3 + 3, color); /* connects */ addedge(ring, ring->vertices, ring->vertices + segs * 2 + 2, color); addedge(ring, ring->vertices + segs, ring->vertices + segs * 3 + 2, color); addedge(ring, ring->vertices + segs + 1, ring->vertices + segs * 3 + 3, color); addedge(ring, ring->vertices + segs * 2 + 1, ring->vertices + segs * 4 + 3, color); reset(s); return ring; } Mesh * createblast(Scene *s, double radius, double density, double seed, int color) { int i; Mesh *blast = addmesh(s); for(i = 0; i < density; ++i) { addline(blast, Pt3d(radius - cos(i) * radius / 2, 0, 0), Pt3d(radius, 0, 0), color); rotateto(s, tan(i) * seed, sin(i) * seed * density, sin(i) * seed + seed); } reset(s); return blast; }
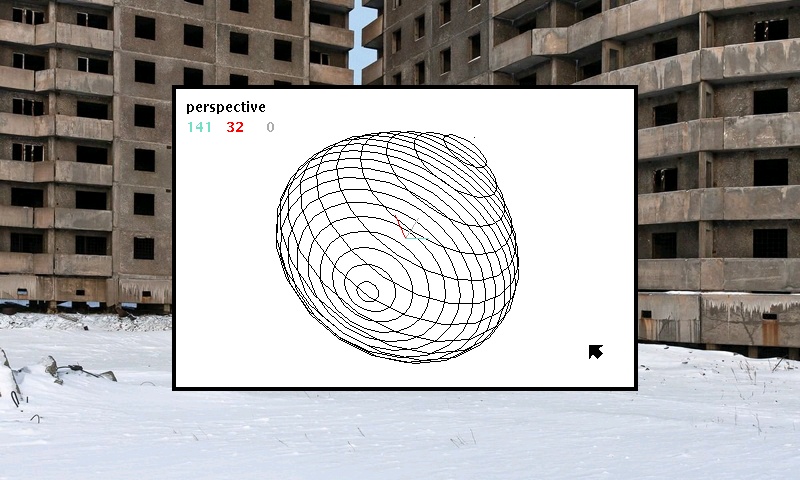
incoming graf3dscene defunct identity visual faqs