10 PRINT "Hello World"
This guide is written specifically for the Varvara implementation of the TinyBASIC compiler, but it should still give a general idea of how to use the language's primitives which are also found in most BASIC implementations.
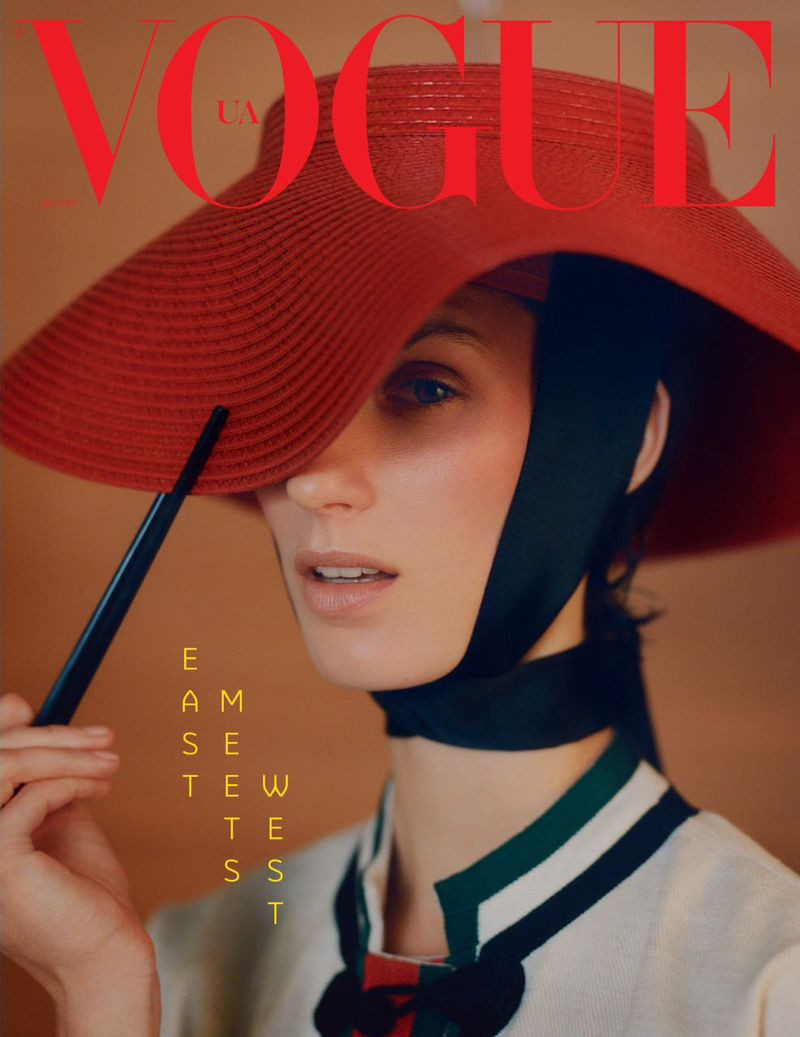
Introduction
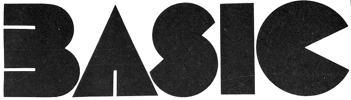
A BASIC program begins with line numbers, control-flow is done by jumping to absolute line numbers which point to compiled BASIC code data. 0 is not a valid line number.
15 LET A=0 20 PRINT "Hello BASIC" 25 LET A=A+1 30 IF A=10 GOTO 50 40 GOTO 20 50 END
Some BASIC compilers will do a light compilation where compiled lines hold a link to the following line in memory, as well as byte opcode for each statement.
40 09 00 0f 80 41 3d 30 00 40 1c 00 14 89 22 48 65 6c 6c 6f 20 42 41 53 49 43 22 00 40 27 00 19 80 41 3d 41 2b 31 00 40 39 00 1e 83 41 3d 31 30 20 47 4f 54 4f 20 35 30 00 40 41 00 28 81 32 30 00 40 47 00 32 8a 00 00 00 00 00 00 00 00 00 00
Expressions
Expressions can include numbers, variables and arithmetic operators. Numbers are decimal, but can be prefixed with $ for hexadecimal, there are no precedence rules. Valid expressions arithmetic operators are +-*/%, bitwise operators are &|, logic operators are <>=!. The smallest number is -32768, the largest number is 32767.
10 LET A=6+7 20 PRINT 52+$20*A 30 END
Variables
Variables are single character uppercased letters of the alphabet. Some variables have special properties, here is the complete list:
- H, reads the screen height, or sets the screen height.
- P, reads a value at address, or sets an address.
- R, reads a random number, or set the random number boundary.
- T, reads the time, in seconds.
- W, reads the screen width, or sets the screen width.
For example, if you wanted to do a program to roll a 6-sided dice using the R special variable.
10 LET R=6, D=R 20 PRINT "You rolled " D+1 "." 30 END
Interaction
The INPUT statement, will halt the execution and wait for an input. This can be used to store a value into a variable. For example, to capture the position of the mouse cursor:
10 PRINT "Touch the screen" 20 INPUT X, Y 30 PRINT "You touched at: " X ", " Y 50 PRINT "Press a button" 60 INPUT B 70 PRINT "You pressed: " B 80 END
To exit an INPUT loop, press the home, or select buttons.
Statements
RUN | Used to begin program execution at the lowest line number. |
---|---|
RUN | |
REM | Permits to add remarks to a program source. |
REM This is a comment. | |
LIST | Causes part or all of the user program to be listed. If no parameters are given, the whole program is listed. A single expression parameter in evaluated to a line number which, if it exists, is listed. |
LIST 200 | |
CLEAR | Formats the user program space, deleting any previous programs. If included in a program the program becomes suicidal when the statement is executed. |
CLEAR | |
INPUT | Halts evaluation, and assigns the result of expressions to each of the variables listed in the argument. Expressions are entered sequencially and separated by a line break, a list of two arguments, will expect two input lines. |
INPUT X, Y | |
LET | Assigns the value of an expression to a variable. |
LET A=123/2, B=$20%2 | |
IF | If the result of the expression is not zero, the statement is executed; if False, the associated statement is skipped. |
IF A>B-2 PRINT "A is greater." | |
PRINT | Prints the values of the expressions and/or the contents of the strings in the console. To print the result of an expression as an ascii character, use '$41, to print the character A. |
PRINT "The result is: " A+B | |
GOTO | Changes the sequence of program execution. |
GOTO 50+A>B | |
GOSUB | Changes the sequence of program execution, and remembers the line number of the GOSUB statement, so that the next occurrence of a RETURN statement will result in execution proceeding from the statement following the GOSUB. |
GOSUB 220 | |
RETURN | Transfers execution control to the line following the most recent unRETURNed GOSUB. If there is no matching GOSUB an error stop occurs. |
RETURN | |
END | Must be the last executable statement in a program. Failure to include an END statement will result in an error stop after the last line of the program is executed. |
END |
Display
There is a handful of routines to draw graphics on the screen, to learn more about the limitations of the display, see the Varvara Screen Specs.
COLOR | Sets the interface RGB colors, see theme. |
---|---|
COLOR $50f2, $b0f9, $a0f8 | |
CLS | Clears the screen. |
CLS | |
DRAW | Sets position of drawing. |
DRAW 100, 320 | |
MODE | Sets drawing mode, see varvara. |
MODE 04 | |
SPRITE | Draws a sprite, uses MODE. |
SPRITE $1c1c, $087f, $0814, $2241 | |
PICT | Draws a picture from a file, uses MODE. |
PICT image10x10.icn |
Memory
Varvara BASIC is able to read and write source files, as well as export compatible roms.
SAVE | Exports your program to example.bas. |
---|---|
SAVE example.bas | |
LOAD | Imports the example.bas program, replaces your current program. |
LOAD example.bas | |
MAKE | Exports your program as a Varvara rom. |
MAKE game.rom | |
POKE | Writes the value of expression B at address A in memory. |
POKE A, B |
incoming 2022