Triplets of ternary digits are encoded in heptavintimal numbers.
There is a need for an encoding akin to hexdecimal for ternary computers. Heptavintimal meets this need, offering a natural encoding for 3-trit trybbles in base 27, or septemvigesimal. It is especially useful for encoding scheme such as TerSCII.
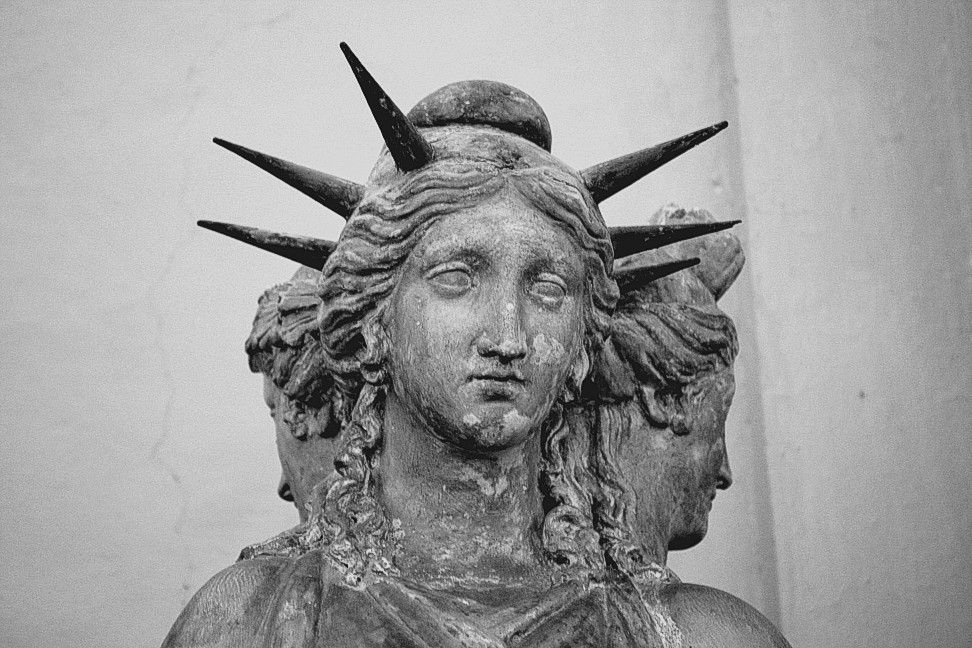
The name heptavintimal is composed of the Greek prefix hepta, meaning seven, followed by the Latin root vinti meaning twenty, with the suffix mal added, to indicate that it is a number base. The mixing of Greek and Latin exactly follows the formation of the word hexadecimal, where the prefix hexi comes from Greek and the root deci is from the Latin.
Bal | -13 | -12 | -11 | -10 | -9 | -8 | -7 | -6 | -5 | -4 | -3 | -2 | -1 | 0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 | 11 | 12 | 13 |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
--- | --0 | --+ | -0- | -00 | -0+ | -+- | -+0 | -++ | 0-- | 0-0 | 0-+ | 00- | 000 | 00+ | 0+- | 0+0 | 0++ | +-- | +-0 | +-+ | +0- | +00 | +0+ | ++- | ++0 | +++ | |
Hept | 0 | A | B | C | D | E | F | G | H | I | J | K | L | M | N | O | P | Q | R | S | T | U | V | W | X | Y | Z |
Uns | 000 | 001 | 002 | 010 | 011 | 012 | 020 | 021 | 022 | 100 | 101 | 102 | 110 | 111 | 112 | 120 | 121 | 122 | 200 | 201 | 202 | 210 | 211 | 212 | 220 | 221 | 222 |
0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 | 11 | 12 | 13 | 14 | 15 | 16 | 17 | 18 | 19 | 20 | 21 | 22 | 23 | 24 | 25 | 26 | |
3's | 0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 | 11 | 12 | 13 | -13 | -12 | -11 | -10 | -9 | -8 | -7 | -6 | -5 | -4 | -3 | -2 | -1 |
The layout used here differs from Douglas W. Jones' proposal which requires a large LUT to render the notation, this one relies on the fact that the 27 characters needed for the system conveniently fits by simply adding a zero at the start of the roman alphabet.
Decimal | Ternary | Nonary | Hept |
---|---|---|---|
1 | 1 | 1 | A |
2 | 2 | 2 | B |
4 | 11 | 4 | D |
8 | 22 | 8 | H |
16 | 121 | 17 | P |
32 | 1012 | 35 | AE |
64 | 2101 | 71 | BJ |
128 | 11202 | 152 | DT |
256 | 100111 | 314 | IM |
512 | 200222 | 628 | RZ |
1024 | 1101221 | 1412 | AJY |
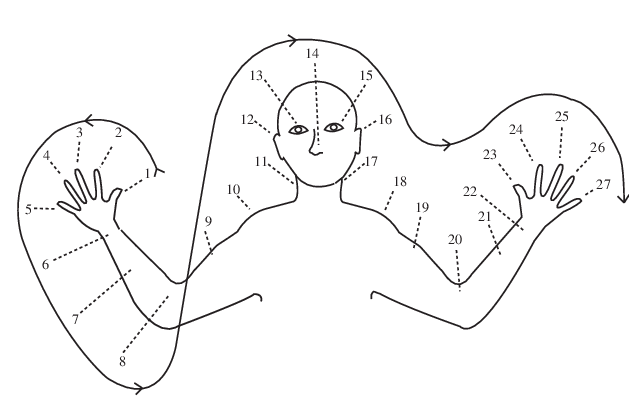
To convert and print the heptavintimal digits of 5 trybbles from a decimal integer stored in a 16-bit address space where each trit takes 2 bits:
typedef uint32_t trint16_t; int ter2bin(trint16_t t) { int sft = 1, acc = 0; while(t) acc += sft * (t & 0x3), t >>= 2, sft *= 3; return acc; } trint16_t bin2ter(int n) { trint16_t sft = 0, acc = 0; while(n > 0) acc |= (n % 3) << sft, n /= 3, sft += 2; return acc; } trint16_t hep2ter(char *str) { char c; trint16_t acc = 0; while((c = *str++)) acc <<= 6, acc |= bin2ter(c ? (c - 'A') + 1 : 0); return acc; } void print_heptavintimal(trint16_t n) { int i; for(i = 4; i > -1; --i) { int t = ter2bin((n >> (i * 6)) & 0x3f); putchar(t ? '@' + t : '0'); } } // heptavintimal to decimal printf("%d", ter2bin(hep2ter("AJY"))); // decimal to heptavintimal print_heptavintimal(bin2ter(1024));
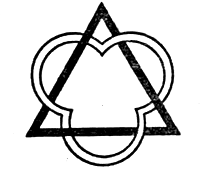
incoming ternary logic