Standard Functions
The following is a collection of implementations for standard functions in Pure Lisp. Predicate functions suffixed with
?
are expressions that return either T or F.
(null? x)
is T if x is NIL, and is F otherwise.(not? x)
, T if x is F, else T.(or? x y)
, T if either arguments are T, else F.(and? x y)
, T if both arguments are T, else F.
(null? λ (x) (EQ x (QUOTE NIL))) (true? λ (x) (IF x (QUOTE T) (QUOTE F))) (not? λ (x) (IF x (QUOTE F) (QUOTE T))) (or? λ (x y) (IF x (QUOTE T) (true? Y))) (and? λ (x y) (IF x (true? y) (QUOTE F))) (number? λ (n) (EQ n (+ n (QUOTE 0))))
List Processing Functions
Length is the number of components in the list ls
.
(length λ (ls) (IF (ATOM ls) (QUOTE 1) (+ (QUOTE 1) (length (CDR ls))) ) )
Member? returns T if the atom e is present in the list ls
.
(member? λ (e ls) (IF (ATOM ls) (QUOTE F) (IF (EQ (CAR ls) e) (QUOTE T) (member? e (CDR ls)))) )
Filter is the list of those components for which the application of
fn
is T.
(filter λ (fn ls) (IF (ATOM ls) (QUOTE NIL) (IF (fn (CAR ls)) (CONS (CAR ls) (filter fn (CDR ls))) (filter fn (CDR ls)) ) ) )
Map is the list whose components are obtained from those of
ls
by application of fn
.
(map λ (fn ls) (IF (ATOM ls) (QUOTE NIL) (CONS (fn (CAR ls)) (map fn (CDR ls))) ) )
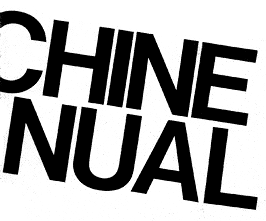